Angular
- 싱글톤
- HTML Tag를 커스텀해서 사용
- Controller로 View의 비즈니스 로직을 만듬
https://docs.angularjs.org/guide/concepts
AngularJS
Loading … There was an error loading this resource. Please try again later.
docs.angularjs.org
AngularJS 사용방법
- html의 head에 angular CDN 추가
1
|
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.7/angular.min.js" data-require="angularjs@1.5.7" data-semver="1.5.7"></script>
|
cs |
- ng ~~ 를 붙여 angular function을 사용
- ng-app : library를 사용하겠다
- ng-init : 초기 세팅값
- ng-controller : View 관리 Controller 선언
- ng-repeat : for문 처럼 반복 출력
- ng-click : click 이벤트 handler
- ng-model : 변수값 handler
사용 결과
HTML
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
<!DOCTYPE html>
<html>
<head>
<link data-require="bootstrap@4.1.3" data-semver="4.1.3" rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" />
<script data-require="bootstrap@4.1.3" data-semver="4.1.3" src="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/js/bootstrap.min.js"></script>
<script data-require="angularjs@1.5.7" data-semver="1.5.7" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.7/angular.min.js"></script>
<link rel="stylesheet" href="style.css" />
<script src="script.js"></script>
</head>
<body ng-app="todo" ng-controller="TodoCtrl">
<div class="container">
<h1>todo list</h1>
<ul class="list-unstyled">
<li ng-repeat="todo in todos | filter:statusFilter">
<div class="input-group mb-3">
<div class="input-group-prepend">
<div class="input-group-text">
<input type="checkbox" aria-label="Checkbox for following text input" ng-model="todo.completed" />
</div>
</div>
<input type="text" class="form-control" aria-label="Text input with checkbox" ng-model="todo.title" />
<div class="input-group-append">
<button class="btn btn-danger" type="button-" id="button-addon2" ng-click="remove(todo)">삭제</button>
</div>
</div>
<date>{{ todo.createdAt | date:'yyyy-MM-dd HH:mm:ss' }}</date>
</li>
</ul>
<button class="btn btn-primary" ng-click="statusFilter={completed:true}">Completed</button>
<button class="btn btn-primary" ng-click="statusFilter={completed:false}">Active</button>
<button class="btn btn-primary" ng-click="statusFilter={}">All</button>
</div>
</body>
</html>
|
cs |
* 삭제버튼 :
remove 함수로 현재 todo 객체 하나를 던진다.
remove 함수에서는 todo 객체가 있는지 findIndex 함수를 이용해서 찾고, 있다면 삭제하는 로직을 작성한다.
* filter :
* ng-click="statusFilter={..." :
Javascript
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
(function() {
var app = angular.module('todo',[]); // angular library function , module 정의
app.controller('TodoCtrl', ['$scope', function($scope){
$scope.todos = [
{
title : 'yoga',
completed:false,
createdAt : Date.now()
},
{
title : 'angular',
completed:false,
createdAt : Date.now()
},
{
title : 'work out',
completed:false,
createdAt : Date.now()
}
];
$scope.remove = function(todo){
// find todo index in todos
var idx = $scope.todos.findIndex(function(item){
return item.id === todo.id;
})
// remove from todos
if(idx > -1 ){
$scope.todos.splice(idx, 1)
}
}
}]);
})();
|
cs |
날짜 포맷 만들기
1
|
<date>{{ todo.createdAt | date:'yyyy-MM-dd HH:mm:ss' }}</date>
|
cs |
출력값 : 2019-05-14 13:24:04
그냥 출력하게 되면 0.01초 단위로 출력된다.
날짜 포맷을 걸어줘야된다.
Angular JS 페이지에 들어가면 포맷 사용방법이 잘 나와있다.
날짜 필터 참고
AngularJS
Loading … There was an error loading this resource. Please try again later.
docs.angularjs.org
사용한 Bootstrap
체크 박스와 텍스트를 좀더 보기좋게 하기 위해서 부트스트랩을 사용했다.
부트스트랩을 사용하기 위해 Javascript에서 부트스트랩 CDN을 추가해주었다.
그리고 필요한 디자인을 아래 부트스트랩에서 찾아 코드를 추가해주고
텍스트 출력이 필요한 부분에 {{ }} 로 변수를 선언해주었다.
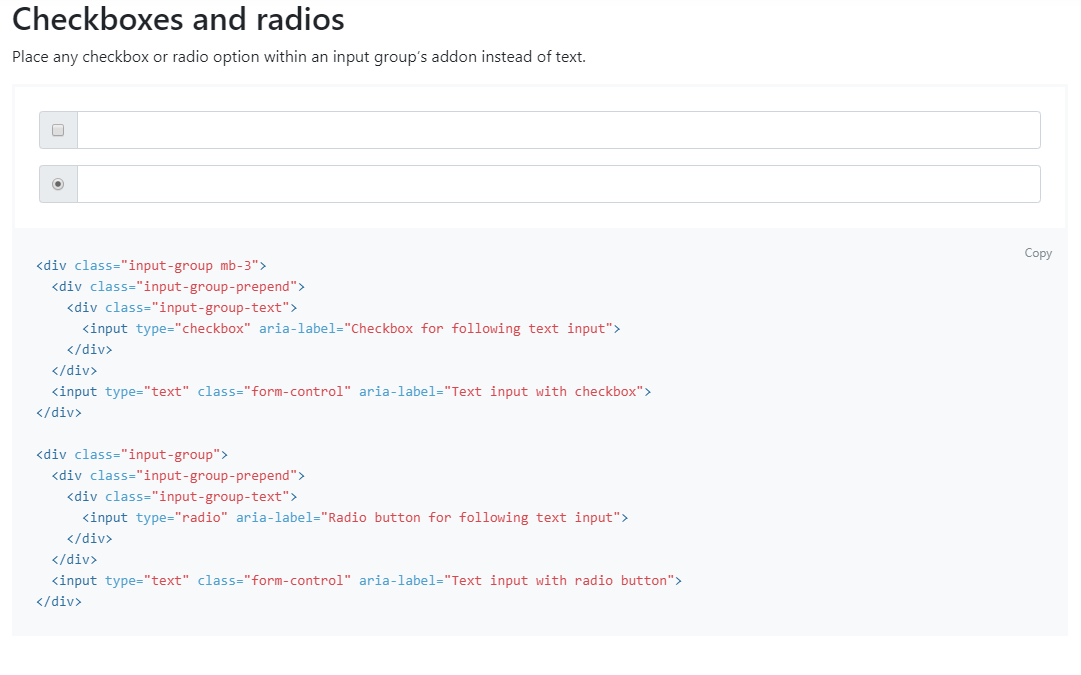
https://getbootstrap.com/docs/4.3/components/input-group/#checkboxes-and-radios
Input group
Easily extend form controls by adding text, buttons, or button groups on either side of textual inputs, custom selects, and custom file inputs.
getbootstrap.com