미리 함수 type을 정의해 놓을 수 있다.
type Add = (a:number, b:number) => number;
const add:Add = (a,b) => a+b;
Overloading
Parameter Type 에 따라서 처리
type Config = { // 객체에 대한 정의
path: string
state: string
}
type Push = { // 함수에 대한 정의
(path: string): void
(config: Config): void
}
const push: Push = (config)=>{
if(typeof config === "string") console.log(config)
else console.log(config.path, config.state)
}
Parameter args 수가 다를때 옵션처리 ( c = option, maybe number )
type Add = {
(a:number, b:number) : number
(a:number, b:number, c:number) : number,
}
const add:Add = (a,b, c?:number) => {
if(c) return a+b+c
else return a+b
}
Polymorphism : 다형성 / 들어올 확실한 타입을 모를때 사용
(Concrete Type : number, string, boolean 처럼 첨에 딱 정의하는 타입)
Generics : Placeholder 에 Typescript 가 알아낸 타입으로 변경해줌
type SuperPrint = {
// (arr: any[]):void
// (arr: (number|boolean|string)[]):void
<TypePlaceholder>(arr: TypePlaceholder[]): void
}
const superPrint : SuperPrint = (arr)=>{
arr.forEach(a=> console.log(a));
}
superPrint([1,true,'string'])
Type : any 로 정의했을 경우 call signatures
Type: generics 로 정의했을 경우 call signatures
Call Signatures
파라미터 타입 정보와 리턴 타입 정보를 보여줌
장점 : 타입 지정과 함수 구현을 분리해서 작성할 수 있음
TypePlaceholder Type으로 받아서 TypePlaceholder Type 으로 Return
type SuperPrintOne = {
<TypePlaceholder>(arr: TypePlaceholder[]): TypePlaceholder
}
const getSuperPrintOne : SuperPrintOne = (arr) => arr[0]
대체로 축약해서 아래와 같이 쓴다.
type SuperPrintT = {
<T>(arr: T[]): T
}
Type 끼리도 상속이 된다.
type User<E> = {
name:string
extraInfo:E
}
type ExtraInfo = {
food:string
}
const user1:User<ExtraInfo>={
name:'dd',
extraInfo:{
food:'dd'
}
}
위 User<ExtraInfo>는 한번더 정의해 줄 수 있다.
type ExtraUser = User<ExtraInfo>;
const user1:ExtraUser={
name:'dd',
extraInfo:{
food:'dd'
}
}
Generics 사용한 Array : number 타입의 array
type A = Array<number>
Generics 사용한 Hooks : number 타입의 state
useState<number>
type Add = (a:number, b:number) => number;
const add:Add = (a,b) => a+b;
Overloading
Parameter Type 에 따라서 처리
type Config = { // 객체에 대한 정의
path: string
state: string
}
type Push = { // 함수에 대한 정의
(path: string): void
(config: Config): void
}
const push: Push = (config)=>{
if(typeof config === "string") console.log(config)
else console.log(config.path, config.state)
}
Parameter args 수가 다를때 옵션처리 ( c = option, maybe number )
type Add = {
(a:number, b:number) : number
(a:number, b:number, c:number) : number,
}
const add:Add = (a,b, c?:number) => {
if(c) return a+b+c
else return a+b
}
Polymorphism : 다형성 / 들어올 확실한 타입을 모를때 사용
(Concrete Type : number, string, boolean 처럼 첨에 딱 정의하는 타입)
Generics : Placeholder 에 Typescript 가 알아낸 타입으로 변경해줌
type SuperPrint = {
// (arr: any[]):void
// (arr: (number|boolean|string)[]):void
<TypePlaceholder>(arr: TypePlaceholder[]): void
}
const superPrint : SuperPrint = (arr)=>{
arr.forEach(a=> console.log(a));
}
superPrint([1,true,'string'])
Type : any 로 정의했을 경우 call signatures
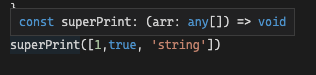
Type: generics 로 정의했을 경우 call signatures

Call Signatures
파라미터 타입 정보와 리턴 타입 정보를 보여줌
장점 : 타입 지정과 함수 구현을 분리해서 작성할 수 있음
TypePlaceholder Type으로 받아서 TypePlaceholder Type 으로 Return
type SuperPrintOne = {
<TypePlaceholder>(arr: TypePlaceholder[]): TypePlaceholder
}
const getSuperPrintOne : SuperPrintOne = (arr) => arr[0]
대체로 축약해서 아래와 같이 쓴다.
type SuperPrintT = {
<T>(arr: T[]): T
}
Type 끼리도 상속이 된다.
type User<E> = {
name:string
extraInfo:E
}
type ExtraInfo = {
food:string
}
const user1:User<ExtraInfo>={
name:'dd',
extraInfo:{
food:'dd'
}
}
위 User<ExtraInfo>는 한번더 정의해 줄 수 있다.
type ExtraUser = User<ExtraInfo>;
const user1:ExtraUser={
name:'dd',
extraInfo:{
food:'dd'
}
}
Generics 사용한 Array : number 타입의 array
type A = Array<number>
Generics 사용한 Hooks : number 타입의 state
useState<number>
'Front-end > Typescript' 카테고리의 다른 글
[TypeScript] Interface (0) | 2022.08.01 |
---|---|
[TypeScript] Class (0) | 2022.08.01 |
[TypeScript] Basic Overview (0) | 2022.07.21 |