부제 : Vue API 모듈 개발시 Axios 모듈만 별도 개발하지 않고 Vuex 에서 개발하는 이유
기반 지식 3줄 요약:
- Vue 에서 사용되는 MVVM(Model-View-ViewModel) 패턴은 ‘이벤트 기반 프로그래밍’ 을 단순화 하기 위해 만든 ‘프레젠테이션 모델 디자인패턴’에서 파생됐다.
- Vue 는 데이터(상태)를 중심으로 움직인다. 데이터(상태)가 바뀌면 ViewModel 객체가 바라보고 있다 감지하여 View를 자동으로 변경한다.
- 어떤 컴포넌트, 어떤 메서드에 의해, 언제 변경되는지 알기 힘들다. 이 때문에 Vuex 를 사용한다.
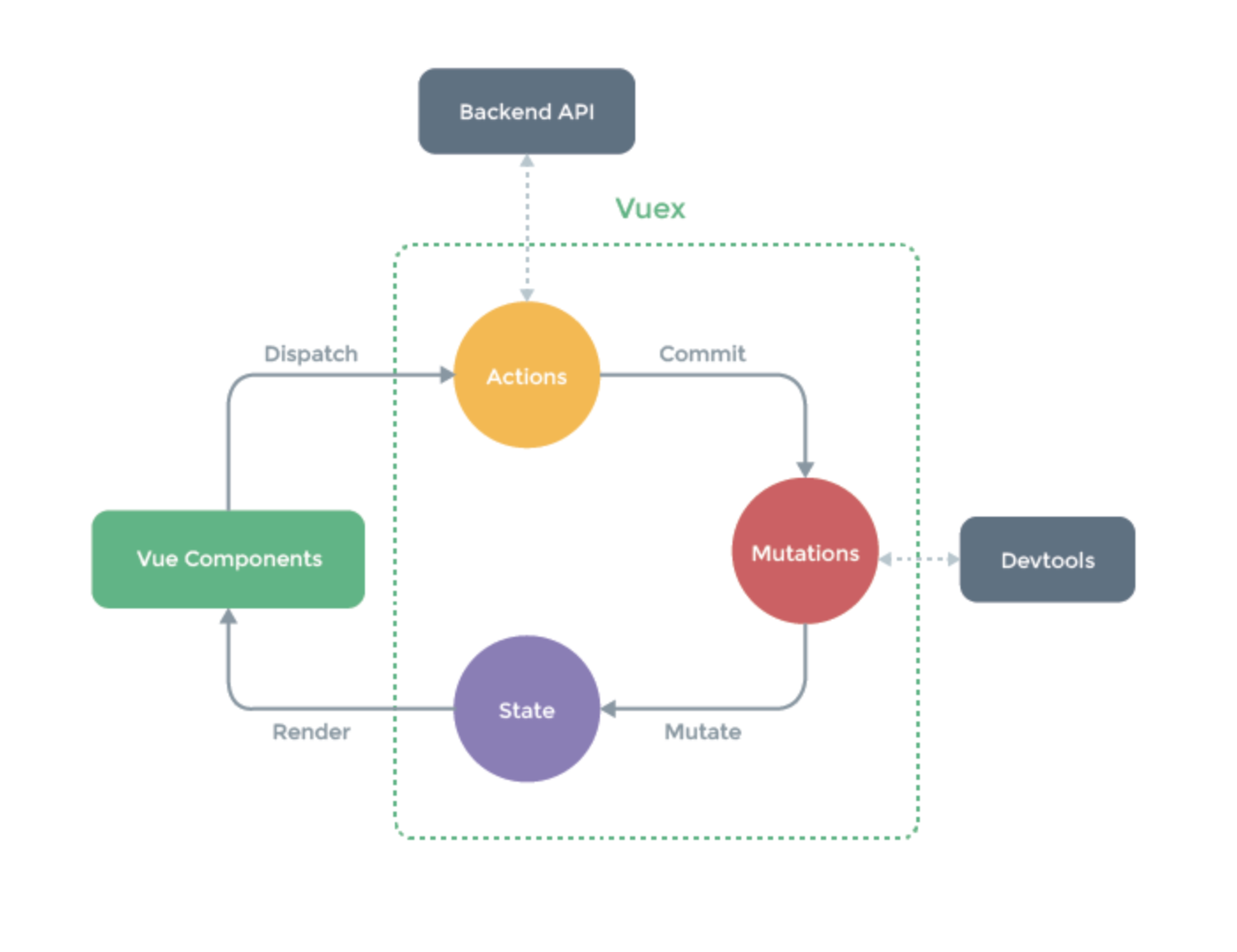
단방향으로 흐르는 Vuex :
- Vue Components 에서 액션 발생 → Action
- API 호출 후 데이터 변이 → Mutation
- 결과 데이터를 받아 상태변경 → State
- 변경된 상태는 Component에 바인딩되어 View 갱신
예시로 분석해보기 :
newTodoItem 을 받아 todoItems 리스트에 추가해주는 예시
const store = new Vuex.Store({
state : {
todoItems; []
},
mutations: {
addItem : (state, newTodoItem) => {
const obj = {completed: false, item: newTodoItem};
state.todoItems.push(obj);
}
}
})
- 단순히 상태를 변화시키는 것이 아니라 Vuex-Mutaions 내부에서 state 사본을 만들어 저장한 후 state 를 변경한다.
- 위 기능을 위해 const store = new Vuex.Store({ }) 처럼 Vuex를 사용해 store를 생성하는 것이다.
- 관리되는 state는 this.$store.state.todoItems 또는 mapState(['todoItems']) 형태로 컴포넌트의 computed 에 바인딩하여 사용한다.
- 컴포넌트 수준에서 상태를 직접 변경하지 않았으면 하기 때문이다.
마지막 줄이 이 글의 부제 : API 모듈 개발시 Axios 모듈만 별도 개발하지 않고 Vuex 에서 개발하는 이유이다.
주의사항 :
- Mutation 은 동기적으로 진행되며 순차적으로 저장된다. Mutation 은 변경의 이력을 남기는 목적이므로 다른 목적으로는 사용하지 않아야한다. (서버통신X, 계산 X) this.$store.commit('addItem', newItem) 과 같이 사용. 마치 DataBase에 적재하는 것 같다. **(TMI : Mutations 안에서 비동기처리(서버통신)을 할 경우 아직 서버통신 콜백 함수가 호출되지 않은 상태에서 스냅샷이 찍히므로 상태변경 이력에 남지 않는다.)
- Action 은 비동기를 지원한다. 서버통신 기다리는 동안 브라우저가 먹통되지 않도록 하기 위함이다.
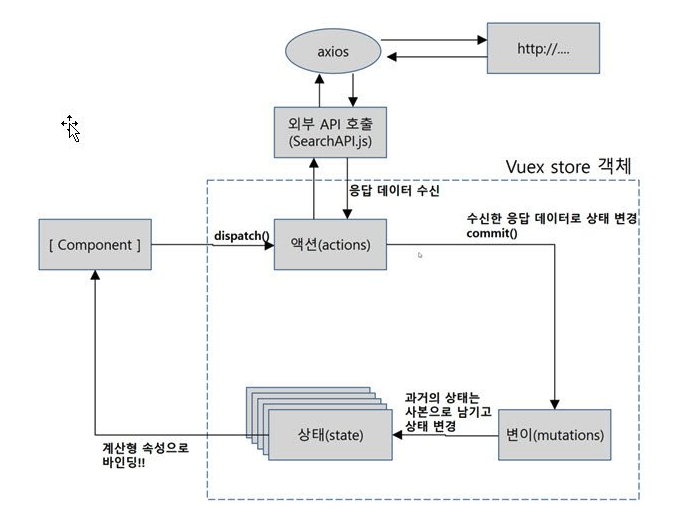
과거 개발 루틴 :
- Back-end가 개발되기 전 Front-end 를 만들기 위해 stub 데이터를 만들어 화면을 구성해야한다.
- Back-end가 개발되고 나면 가짜 로직 및 데이터를 들어내는 리팩토링 작업 필요하다.
- api/index.js 에 axios 전부 선언 및 export에 선언해야 한다. → 이 방법은 API 함수 및 데이터를 바로 컴포넌트에 뿌려주는 대신 import로 index.js 를 불러와서 함수 등록을 해주어야함
<scirpt>
import { fetchAPI } from '../api/index.js';
export default {
data() {
return {
asks : []
}
},
created() {
var vm = this;
fetchAPI().then(res => {
vm.asks = res.data;
})
.catch(err => {
console.log(err);
}
}
}
</script>
Vuex 사용 루틴 :
- Front-end 와 Back-end 완벽분리가 가능하다.
- API가 없더라도 Action을 통해 중첩된 API 호출 선 개발이 가능하다.
- API Return 데이터 변경이 있더라도 각 컴포넌트에서 수정하는 것이 아닌 Vuex에서 일괄 수정이 가능하다.
- Computed 에 변수마다 코딩해주는 대신 Vuex 헬퍼 함수(mapGetters)를 사용하여 코딩 양을 줄일 수 있다.
Helper - mapGetters :
- 상태 연산이 여러 컴포넌트에 반복적으로 사용되는 경우 필요하다. (필수X)
- 자주 처리해야 하는 상태값을 캐싱하여 코드의 반복을 줄이고 성능과 가독성을 높인다.this.$store.getters.doneTodosCount ← property 형으로 사용될 때 캐싱됨this.$store.getters.doneTodosCount(todoLIst) ← method 형으로 사용될 때는 캐싱되지 않음
사용전
App.vue
computed:{
doneTodosCount(){
return this.$store.state.todoItems.filter(todo => todo.done).length;
}
}
Child.vue
computed:{
doneTodosCount(){
return this.$store.state.todoItems.filter(todo => todo.done).length;
},
anotherParam(){...}
}
사용후
store.js
getters: {
doneTodosCount(){
return this.state.todoItems.filter(todo => todo.done).length;
}
}
App.vue
computed: {
...mapGetters([
'doneTodosCount'
])
}
Child.vue
computed: {
...mapGetters([
'doneTodosCount'
]),
anotherParam(){...}
}
Reference :
https://medium.com/vue-mastery/vuex-explained-visually-f17c8c76d6c4
Vuex Explained Visually
Managing state in an application full of components can be difficult. Facebook discovered this the hard way and created the Flux pattern…
medium.com
https://hagohobby.tistory.com/19
[vue.js] Vuex를 이용한 데이터 처리
요즘 계속해서 일이 끝난 후 한~두시간씩 캡틴판교님의 강의를 보면서 vue.js를 익히고 있다. 비동기 처리, Promise, this 등 자바하고는 다른 개념이 많아서 처음엔 이해 안가는 부분들이 많았는데
hagohobby.tistory.com
https://zendev.com/2018/05/21/vuex-perfect-interface-frontend-backend.html
Why VueX Is The Perfect Interface Between Frontend and API
The increase in complexity in front end web development has driven to increasing amounts of specialization and separation of front and back end. This specialization and increased complexity has a number of benefits - the quality of user experiences on the
zendev.com
https://steemit.com/kr/@stepanowon/vuex
Vuex를 사용해야 할 이유? — Steemit
Vue를 사용해야 할 이유? 이 문서를 읽기 전에... 목차 왜 Vuex를 사용하는가? 상태(state)와 변이(mutations) 게터(Getters) 액션(Actions) 대규모 애플리케이션의 저장소 왜 Vuex를 왜 사용하는가 Vue를 공부하
steemit.com
'Front-end' 카테고리의 다른 글
Storybook (0) | 2023.02.15 |
---|---|
깃헙 웹페이지 올리기 github.io (0) | 2021.09.08 |